ChatGPT Clone
A ChatGPT clone built with Next.js and Vercel AI SDK.
- Next.js
- React
- TypeScript
- OpenAI
- PostgreSQL
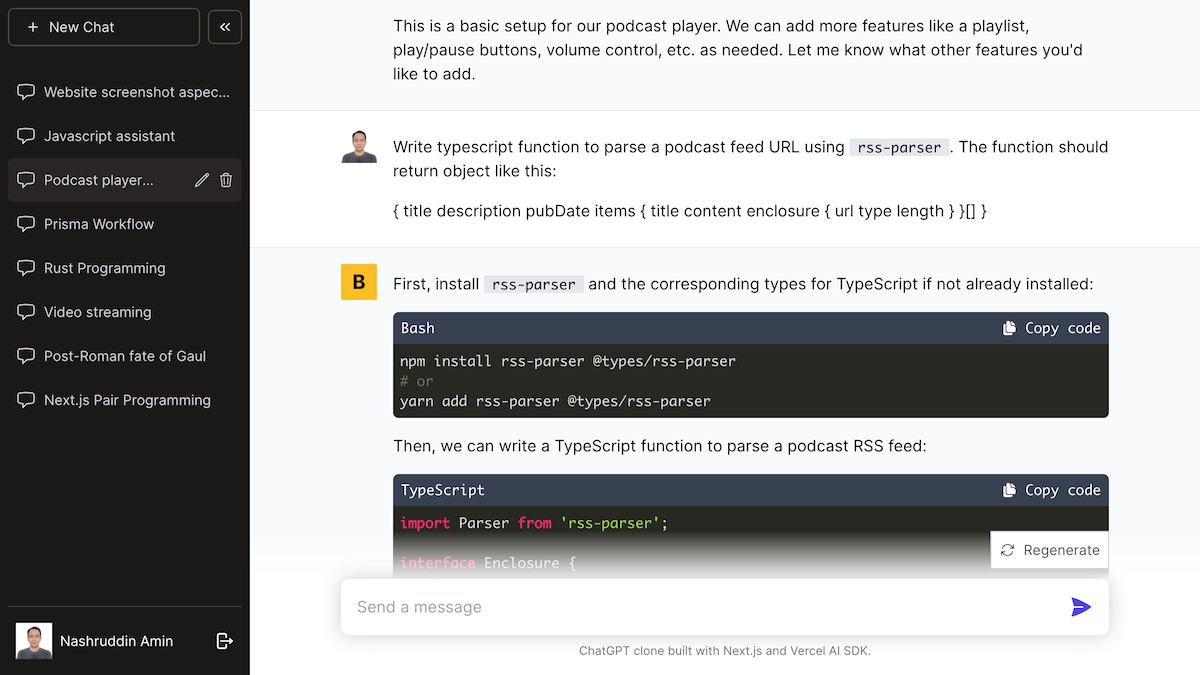
About this project
This is a full stack application with similar functionality as the original ChatGPT, built with Next.js and the Vercel AI SDK. I was really impressed with the original ChatGPT user interface. In particular, I really love the streaming text responses from the LLM model. The user interface and the functionality really motivated me to build a ChatGPT clone myself.
One of the features that I wanted to have in this ChatGPT clone was to allow users to edit the chat messages. Editing the user messages allows users to refine their inptus. And by allowing the users to edit the response messages, they can fix any hallucination or incorrect responses returned by the model.
Another feature that I thought important is the ability to delete existing messages that are no longer needed. This will keep the conversation short and will reduce the costs when calling the OpenAI API.
How it works
At its core, the app is calling the Chat Completions API using the Vercel AI SDK.
// actions.ts
import OpenAI from 'openai'
const openai = new OpenAI({ ... })
...
export async function POST(request: Request) {
const { messages } = await request.json()
try {
const chatCompletion = await openai.chat.completions.create({
model: 'gpt-3.5-turbo-16k',
temperature: 0.7,
stream: true,
messages
})
const stream = OpenAIStream(chatCompletion)
return new StreamingTextResponse(stream)
} catch (err) {
return NextResponse.json({
error: `Error communicating wih OpenAI: ${err}`
}, { status: 500 })
}
}
Streaming UI will make the app more seamless, as it can display part of the response as they become available.
The React frontend is responsible for handling user input as well as displaying the streaming responses. To make the chat persistent, they are saved to a PostgreSQL database using the Prisma ORM.
Demo and download
I deployed this project on Vercel and you can try out the demo. The source code is also available on Github.